本篇文章带你了解如何创建微信小程序中的自定义组件
step1:初始化
初始化一个小程序,删掉里面的示例代码,并新建立一个components
文件夹,这个文件夹是用于存放我们以后开发时用到的组件。
本次开发,是实现一个弹窗组件
我们在components
文件夹新建一个components
,名字你随意起啦~我这里就写Dialog了~
他就会生成相应的 json wxml wxss js
4个文件
step2:画出页面
创建完组件之后,接下来就是组件相关的配置了,也就是将 dialog.json
中 component
字段设为 true
代码:
{ "component": true,// 自定义组件声明 "usingComponents": {}// 可选,用于引用别的组件 }
然后就是编写wxml和wxss,不多BB,直接贴代码
wxml:
<view class='wx_dialog_container' hidden="{{!isShow}}"> <view class='wx-mask'></view> <view class='wx-dialog'> <view class='wx-dialog-title'>{{ title }}</view> <view class='wx-dialog-content'>{{ content }}</view> <view class='wx-dialog-footer'> <view class='wx-dialog-btn' catchtap='_cancelEvent'>{{ cancelText }}</view> <view class='wx-dialog-btn' catchtap='_confirmEvent'>{{ confirmText }}</view> </view> </view> </view>
wxss:
.wx-mask{ position: fixed; z-index: 1000; top: 0; right: 0; left: 0; bottom: 0; background: rgba(0, 0, 0, 0.3); } .wx-dialog{ position: fixed; z-index: 5000; width: 80%; max-width: 600rpx; top: 50%; left: 50%; -webkit-transform: translate(-50%, -50%); transform: translate(-50%, -50%); background-color: #FFFFFF; text-align: center; border-radius: 3px; overflow: hidden; } .wx-dialog-title{ font-size: 18px; padding: 15px 15px 5px; } .wx-dialog-content{ padding: 15px 15px 5px; min-height: 40px; font-size: 16px; line-height: 1.3; word-wrap: break-word; word-break: break-all; color: #999999; } .wx-dialog-footer{ display: flex; align-items: center; position: relative; line-height: 45px; font-size: 17px; } .wx-dialog-footer::before{ content: ''; position: absolute; left: 0; top: 0; right: 0; height: 1px; border-top: 1px solid #D5D5D6; color: #D5D5D6; -webkit-transform-origin: 0 0; transform-origin: 0 0; -webkit-transform: scaleY(0.5); transform: scaleY(0.5); } .wx-dialog-btn{ display: block; -webkit-flex: 1; flex: 1; -webkit-tap-highlight-color: rgba(0, 0, 0, 0); position: relative; } .wx-dialog-footer .wx-dialog-btn:nth-of-type(1){ color: #353535; } .wx-dialog-footer .wx-dialog-btn:nth-of-type(2){ color: #3CC51F; } .wx-dialog-footer .wx-dialog-btn:nth-of-type(2):after{ content: " "; position: absolute; left: 0; top: 0; width: 1px; bottom: 0; border-left: 1px solid #D5D5D6; color: #D5D5D6; -webkit-transform-origin: 0 0; transform-origin: 0 0; -webkit-transform: scaleX(0.5); transform: scaleX(0.5); }
step3:编写逻辑
页面已经画好了,现在需要使用js去给页面中的{{isShow}},{{title}}之类的变量去赋值
js:
Component({ options: { multipleSlots: true // 在组件定义时的选项中启用多slot支持 }, /** * 组件的属性列表 * 用于组件自定义设置 */ properties: { // 弹窗标题 title: { // 属性名 type: String, // 类型(必填),目前接受的类型包括:String, Number, Boolean, Object, Array, null(表示任意类型) value: '标题' // 属性初始值(可选),如果未指定则会根据类型选择一个 }, // 弹窗内容 content :{ type : String , value : '弹窗内容' }, // 弹窗取消按钮文字 cancelText :{ type : String , value : '取消' }, // 弹窗确认按钮文字 confirmText :{ type : String , value : '确定' } }, /** * 私有数据,组件的初始数据 * 可用于模版渲染 */ data: { // 弹窗显示控制 isShow:false }, /** * 组件的方法列表 * 更新属性和数据的方法与更新页面数据的方法类似 */ methods: { /* * 公有方法 */ //隐藏弹框 hideDialog(){ this.setData({ isShow: !this.data.isShow }) }, //展示弹框 showDialog(){ this.setData({ isShow: !this.data.isShow }) }, /* * 内部私有方法建议以下划线开头 * triggerEvent 用于触发事件 */ _cancelEvent(){ //触发取消回调 this.triggerEvent("cancelEvent") }, _confirmEvent(){ //触发成功回调 this.triggerEvent("confirmEvent"); } } })
step4:引用
在index.json里面引入组件
{ "usingComponents": { "dialog": "/components/Dialog/dialog" } }
在index.wxml里面引入这个组件,并添加自定义的值
<dialog id='dialog' title='标题啊标题' content='我是里面的文本' cancelText='取消按钮' confirmText ='确定按钮' bind:cancelEvent="cancel_Click" bind:confirmEvent="cancel_Click"></dialog>
在index.js添加组件相关绑定回调
const app = getApp()Page({ /** * 生命周期函数--监听页面初次渲染完成 */ onReady: function () { //获得dialog组件 this.dialog = this.selectComponent("#dialog");//你也可以设置class,那么就是.classname和CSS选择器是一样的 }, showDialog(){ this.dialog.showDialog(); }, //取消事件 cancel_Click(){ console.log('你点击了取消'); this.dialog.hideDialog(); }, //确认事件 cancel_Click(){ console.log('你点击了确定'); this.dialog.hideDialog(); } })
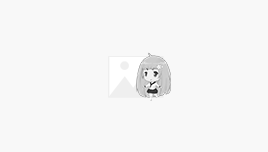
微信扫码查看本文
发表评论